How To Convert A List Into A Set In Python?
To convert a list into a set in Python, you can use the built-in set() function. A Set is an unordered collection of data type items that are immutable (cannot be changed). This means that once created, no item within the Set can be modified or replaced.
The syntax for converting a list to a set is:
set(list_name) For example:
original_list = [1, 2, 3] # Defining original list
new_set = set(original_list) # Converting to set print(new_set) # Output: {1, 2, 3}
Alternatively if you have duplicate values present in your list and need to remove them before conversion then use the following syntax instead: new_set = set(dict.fromkeys(originalList)) The converted Set will contain only unique values from the original List without any duplicates.
- Create a list: The first step in converting a list into a set is to create the initial list. This can be done by assigning values to an array variable using square brackets, e: myList = [1,2,3].
- Use Set() Method: To convert the list into a set, use the built-in function ‘set’ with your list as an argument for it, e: mySet = set(myList). This will return a new set object which contains all of the unique elements from your original list.
- Print Your New Set: Once you have converted your list into a set you can simply print out its contents using either Python’s ‘print’ or ‘pprint’ functions (the latter is used when dealing with complex data structures) and view the result in real time on screen or within IDEs such as PyCharm and Spyder etc: print(mySet).
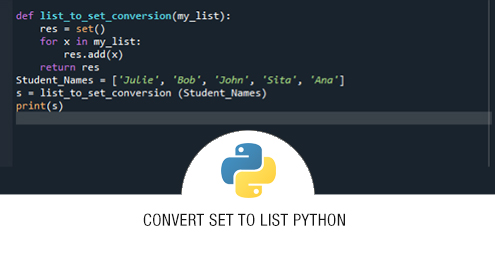
Credit: linuxhint.com
Can You Turn a List of Lists into a Set in Python?
Yes, you can turn a list of lists into a set in Python. To do so, you must first use the ‘set’ function to convert each individual sub-list into its own set. You then combine these sets using the union operator | and wrap them all in another call to the ‘set’ function.
This will produce a new set that contains all of the items from the original list of lists. Keep in mind that since sets are unordered collections any duplicate elements will be removed during this process.
Can You Convert a List to a Set?
Yes, it is possible to convert a list to a set. A set is an unordered collection of unique elements, which means that any element in the set must be distinct from all other elements; this eliminates duplication and ensures that no two elements have the same value. To convert a list into a set, you can use the Python built-in function ‘set()’.
This function takes one argument (the list) and returns a new set object with only the unique values from the original list. Additionally, if you need to maintain order in your data structure after conversion, you may consider using collections module’s OrderedSet instead of regular sets for better performance when dealing with large datasets.
How to Convert a List of Lists to Set of Lists in Python?
To convert a list of lists to a set of lists in Python, you can use the built-in set() function. This function takes any iterable and converts it into an unordered collection without duplicate elements. To convert a list of lists to a set of lists, simply pass each inner list as an argument to the set() function and then assign the result back to your original variable.
What is Set () in Python?
The set() function in Python is a built-in data type that allows you to store collections of unique elements. The elements inside the set can be any type such as strings, numbers, tuples or even other sets. It is an unordered collection and does not allow duplicate entries.
Sets are useful for eliminating duplicates from a sequence or list and also for performing mathematical operations such as intersection, union, difference etc., on multiple sets.
Convert list into a string in python | convert list into a set in python | list into a tuple
Convert List to Set Python Unhashable Type ‘List’
The concept of converting a list to a set in Python may seem simple enough, however it is not possible due to the inherent nature of lists. A list has an unhashable type which cannot be converted into a set as sets are based on hash tables and require all items within them to be immutable (unchangeable). In order for one to convert a list into a set, they need to first create an immutable object from each item in the original list.
This can then be used as input when constructing the new set.
Convert List to Set Python Time Complexity
The time complexity of converting a list to a set in Python is O(n). This means that the time taken for conversion grows linearly with the size of the list. The advantage of using sets over lists is that it eliminates duplicates, and also allows faster lookup operations since it uses hash tables internally to store its elements.
Convert List to Set Java
Java has a convenient way of converting lists to sets. A Set is an unordered collection of unique elements, meaning that no two elements can be the same. To convert a List to a Set, you first need to create an empty instance of the desired Set type, then use its addAll() method and pass in your list as an argument.
This will populate the set with all values from the list while removing duplicate entries automatically.
Convert List to Set Without Changing Order Python
Using Python, it is possible to convert a list into a set without changing its order. This can be accomplished by using the tuple syntax and the set method, which will take in any iterable object as an argument. The result of this conversion would be that all duplicate elements are removed from the list while still maintaining their original order.
Conclusion
In conclusion, converting a list into a set in Python is an extremely useful and versatile skill. It can simplify complex data structures, reduce memory usage and improve performance by removing duplicate elements from lists. Additionally, using sets provides powerful methods for performing set operations such as intersection and union.
With the knowledge outlined in this blog post, you now have the necessary tools to convert any list into a set in Python with ease.