How to Have an Input Change the Dimensions Python?
Incorporating user inputs to modify dimensions in Python is a highly useful technique, particularly in applications involving data manipulation, image processing, and GUI development. This article will provide a comprehensive guide on how to dynamically change dimensions based on user input using Python, with practical examples from different applications.
1. Changing Array Dimensions with NumPy:
NumPy, a core library for numerical computing in Python, offers tools for handling arrays and matrices. To change the dimensions of an array based on user input, you can use the reshape()
function.
Here’s an example:
import numpy as np
# User specifies new dimensions
rows = int(input("Enter the number of rows: "))
cols = int(input("Enter the number of columns: "))
# Original array
original_array = np.arange(10) # An array of ten elements
print("Original Array:", original_array)
# Reshape array based on user input
if rows * cols == len(original_array):
reshaped_array = original_array.reshape(rows, cols)
print("Reshaped Array:\n", reshaped_array)
else:
print("Error: The product of rows and columns must equal the total elements in the array.")
This code snippet collects the desired dimensions from the user and reshapes the array accordingly, provided that the total number of elements remains constant.
2. Modifying Image Dimensions with PIL:
The Python Imaging Library (PIL), now known as Pillow, is essential for image processing tasks.
To resize an image based on user input:
from PIL import Image
# Load an image
image = Image.open("sample.jpg")
# User defines the new size
new_width = int(input("Enter the new width: "))
new_height = int(input("Enter the new height: "))
# Resize the image
resized_image = image.resize((new_width, new_height))
resized_image.show()
This example prompts the user for new dimensions and resizes the image accordingly. It’s a straightforward way to manipulate image sizes dynamically.
3. Adjusting Widget Dimensions in Tkinter:
Tkinter, a standard GUI library for Python, allows the creation of interfaces.
Here’s how you can change the dimensions of a widget like a window based on user input:
import tkinter as tk
# Create the main window
root = tk.Tk()
root.title("Resize Window")
# Function to resize
def resize():
new_width = int(width_entry.get())
new_height = int(height_entry.get())
root.geometry(f"{new_width}x{new_height}")
# User input fields
width_entry = tk.Entry(root)
width_entry.pack()
height_entry = tk.Entry(root)
height_entry.pack()
# Button to trigger resize
resize_button = tk.Button(root, text="Resize", command=resize)
resize_button.pack()
# Start the GUI loop
root.mainloop()
This code snippet creates a simple GUI where the user can enter new dimensions, and the main window will resize accordingly.
Conclusion
The ability to dynamically adjust dimensions using Python is powerful across various fields such as scientific computing, web development, and data analysis. By leveraging libraries like NumPy for arrays, Pillow for images, and Tkinter for GUI elements, Python programmers can effectively build applications that respond to user inputs in real time, enhancing interactivity and usability.
FAQs
How to add a new dimension to a NumPy array?
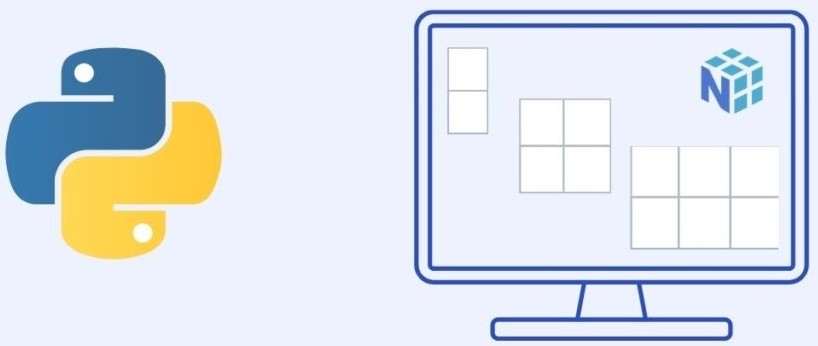
To add a new dimension to a NumPy array, you can use several methods such as np.newaxis
, np.expand_dims()
, and np.reshape()
. Here’s a summary based on the provided sources:
- Using
np.newaxis
:np.newaxis
is an alias forNone
and adds a new dimension of size 1 at the specified position within the array.- Example:
a[:, :, np.newaxis]
adds a new dimension to a 2D arraya
. - This method is useful for controlling broadcasting and simplifying array manipulation.
- Using
np.expand_dims()
:np.expand_dims()
function adds a new dimension to the array at the specified position.- Example:
np.expand_dims(a, 0)
adds a new dimension at the beginning of the arraya
. - This method provides an intuitive way to increase the dimensionality of an array.
- Using
np.reshape()
:- The
np.reshape()
function or thereshape()
method of an ndarray can change the shape of an array. - Example:
a.reshape(1, 2, 3)
reshapes a 2D arraya
into a 3D array. - This method allows you to transform arrays to any desired shape, adding dimensions as needed.
- The
These methods offer flexibility in manipulating the dimensions of NumPy arrays, which is essential for various data processing tasks, including machine learning and scientific computing.
How can I reshape a NumPy array to a desired shape?
You can use the np.reshape()
function or the reshape()
method of an ndarray
to change the shape of an array. This method allows you to transform arrays to any desired shape, adding dimensions as needed.
How can I remove a dimension of size 1 in a NumPy array?
You can use the np.squeeze()
function to remove any dimensions of size 1 from the array. This function is useful for removing unnecessary dimensions and simplifying array manipulation.